how to make Rock,Paper,Scissors in c# console Application
HOW TO SEARCH ANYONE ON TWITTER USING TWITTER SEARCH ENGINE ON C# CONSOLE APPLICATION
NOTE:
- YOU NEED TO HAVE MICROSOFT VISUAL STUDIO (2010 OR NEWER VERSION) OR YOU CAN HAVE ANY PLATFORM WHICH SUPPORTS C# CONSOLE APPLICATION.
- AFTER CREATING THE PROJECT, FIRST ADD THE LIBRARIES:
- using System.Net;
- using System.IO;
- using System.Diagnostics;
- using System.Threading;
NOW,
Write or paste the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ROCKPAPERSCISSORS
{
class Program
{
static void Main(string[] args)
{
string inputPlayer, inputCPU = null;
int randomInt;
bool playAgain = true;
while (playAgain)
{
int scorePlayer = 0;
int scoreCPU = 0;
while (scorePlayer < 3 && scoreCPU < 3)
{
Console.Write("Choose between ROCK, PAPER and SCISSORS: ");
inputPlayer = Console.ReadLine();
inputPlayer = inputPlayer.ToUpper();
Random rnd = new Random();
randomInt = rnd.Next(1, 4);
switch (randomInt)
{
case 1:
inputCPU = "ROCK";
Console.WriteLine("Computer chose ROCK");
if (inputPlayer == "ROCK")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "PAPER")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
else if (inputPlayer == "SCISSORS")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
break;
case 2:
inputCPU = "PAPER";
Console.WriteLine("Computer chose PAPER");
if (inputPlayer == "PAPER")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "ROCK")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
else if (inputPlayer == "SCISSORS")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
break;
case 3:
inputCPU = "SCISSORS";
Console.WriteLine("Computer chose SCISSORS");
if (inputPlayer == "SCISSORS")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "ROCK")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
else if (inputPlayer == "PAPER")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
break;
default:
Console.WriteLine("Invalid entry!");
break;
}
Console.WriteLine("\n\nSCORES:\tPLAYER:\t{0}\tCPU:\t{1}", scorePlayer, scoreCPU);
}
if (scorePlayer == 3)
{
Console.WriteLine("Player WON!");
}
else if (scoreCPU == 3)
{
Console.WriteLine("CPU WON!");
}
else
{
}
Console.WriteLine("Do you want to play again?(y/n)");
string loop = Console.ReadLine();
if (loop == "y")
{
playAgain = true;
Console.Clear();
}
else if (loop == "n")
{
playAgain = false;
}
else
{
}
}
}
}
}
The output will be :
*)It'll run until CPU or User get 3 points,whoever gets 3 points first will win the match.
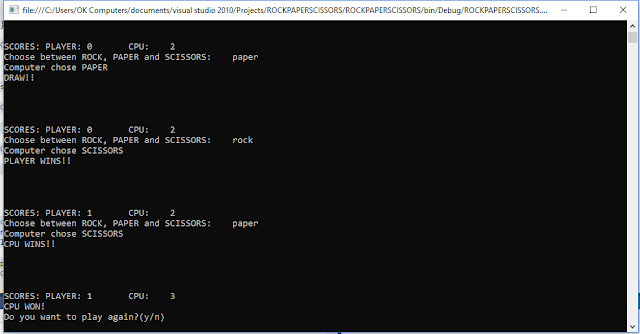
Write or paste the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ROCKPAPERSCISSORS
{
class Program
{
static void Main(string[] args)
{
string inputPlayer, inputCPU = null;
int randomInt;
bool playAgain = true;
while (playAgain)
{
int scorePlayer = 0;
int scoreCPU = 0;
while (scorePlayer < 3 && scoreCPU < 3)
{
Console.Write("Choose between ROCK, PAPER and SCISSORS: ");
inputPlayer = Console.ReadLine();
inputPlayer = inputPlayer.ToUpper();
Random rnd = new Random();
randomInt = rnd.Next(1, 4);
switch (randomInt)
{
case 1:
inputCPU = "ROCK";
Console.WriteLine("Computer chose ROCK");
if (inputPlayer == "ROCK")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "PAPER")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
else if (inputPlayer == "SCISSORS")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
break;
case 2:
inputCPU = "PAPER";
Console.WriteLine("Computer chose PAPER");
if (inputPlayer == "PAPER")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "ROCK")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
else if (inputPlayer == "SCISSORS")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
break;
case 3:
inputCPU = "SCISSORS";
Console.WriteLine("Computer chose SCISSORS");
if (inputPlayer == "SCISSORS")
{
Console.WriteLine("DRAW!!\n\n");
}
else if (inputPlayer == "ROCK")
{
Console.WriteLine("PLAYER WINS!!\n\n");
scorePlayer++;
}
else if (inputPlayer == "PAPER")
{
Console.WriteLine("CPU WINS!!\n\n");
scoreCPU++;
}
break;
default:
Console.WriteLine("Invalid entry!");
break;
}
Console.WriteLine("\n\nSCORES:\tPLAYER:\t{0}\tCPU:\t{1}", scorePlayer, scoreCPU);
}
if (scorePlayer == 3)
{
Console.WriteLine("Player WON!");
}
else if (scoreCPU == 3)
{
Console.WriteLine("CPU WON!");
}
else
{
}
Console.WriteLine("Do you want to play again?(y/n)");
string loop = Console.ReadLine();
if (loop == "y")
{
playAgain = true;
Console.Clear();
}
else if (loop == "n")
{
playAgain = false;
}
else
{
}
}
}
}
}
The output will be :
*)It'll run until CPU or User get 3 points,whoever gets 3 points first will win the match.
Comments
Post a Comment